Unit Testing And How To Do A Unit Test
Individual units or software components are tested in unit testing, which is a sort of software testing. The goal is to authenticate that each unit of software code works as intended. Developers carry out unit testing throughout an application’s development (coding) phase. Advanced unit testing isolates a section of code and ensures that it is correct. An individual functionality, method, procedure, module, or object can be considered a unit. Unit tests are the initial level of testing performed before Integration tests in the V Model, SDLC, and STLC. Unit testing is a kind of White Box testing often done by the software developer. Because of time constraints or developers’ reluctance to test in the real world, Quality assurance engineers (QA engineers) also perform unit testing.
Table on content:
- How to do Unit Testing
- Unit Testing Techniques
- Unit Testing Example: Mock Objects
- Unit Testing Tools
- Test-Driven Development (TDD) & Unit Testing
- Why Unit Testing?
- Unit Testing Myth
- Advantages of Unit Testing
- Disadvantages of Unit Testing
- Unit Testing Best Practices
- Summary
How To Do Unit Testing
UnitTest is a framework developers utilize to create and design automated test cases for unit testing. Software developers write a portion of code to test a specific function in a software application as part of Unit Testing. Developers can isolate this functional test more thoroughly, revealing any redundant dependencies between the tested functions and other units, which can then be discarded.
Two Types of Unit Testing
- Manual
- Automated
Unit testing is frequently automated, although it can also be done manually. However, software engineering has no preference for one over the other; test automation is desirable. A step-by-step instructional document may be used in a manual method to unit testing. Under the automated approach:
- A developer writes a piece of code in the program only to test the function. When the program is introduced, they will comment out and eventually eliminate the test code.
- A developer could potentially isolate the function to test it thoroughly. This more advanced unit testing method entails copying and pasting code into its testing environment rather than the natural one. Isolating the code helps in the discovery of unneeded dependencies between the code under test and other units/data spaces in the product. These reliance’s can then be eliminated.
- To create automated test cases, most programmers employ the UnitTest Framework. The developer uses an automation framework to code criteria into the test to ensure correct code. The framework logs failing unit test cases while they are being executed. Many frameworks will automatically highlight and report these failed test cases in a summary format. The framework can halt subsequent testing depending on the severity of a failure.
- The Unit Testing workflow is as follows:
- Create Test Cases
- Examine/Revise
- Establish a baseline
- Execute test cases.
Unit Testing Techniques
Unit testing strategies are divided into three categories:
- Black-box testing
- White-box testing
- Gray-box testing
Black-box testing | Black-box testing, which involves testing the user interface as well as input and output. |
White-box testing | White box testing, which consists in testing the software application’s functional behavior |
Gray-box testing | Gray box testing, which involves executing test suites, test methods, test cases, and risk analysis. |
The following are a few of the code coverage strategies used in unit testing:
- Statement Coverage
- Decision Coverage
- Branch Coverage
- Condition Coverage
- Finite State Machine Coverage
Unit Test Example: Mock Objects
Mock objects are used in unit testing to test code that isn’t yet part of a complete application—mock objects stand in for the missing aspects of the program. You might have a function that requires production environment variables/ objects that are yet to be generated. Those functions will be submitted for unit testing in the form of mock objects created specifically for that code area’s unit testing.
Unit Testing Tools
To aid with unit testing, there is a variety of automated unit test software available. We’ll give you some samples below:
- Junit: Junit is a free testing tool for the Java programming language. It includes assertions that can be used to distinguish between test methods. Before entering data into the code, this utility tests it.
- NUnit: NUnit is a well-known unit-testing framework for all.NET languages. It is an open-source program that allows users to write scripts manually. It allows you to execute data-driven tests in parallel.
- JMockit: It is an open-source unit testing software. It is a code coverage tool that includes measurements (metrics) for lines and paths. It has recording and verification syntax for copying APIs (API method). Line coverage, Data coverage and Path coverage are all accessible with this tool.
- EMMA: EMMA is an open-source toolset for evaluating and reporting Java code.EMMA is Java-based; therefore, there are no external library dependencies, and the source code is available. Method, block and line are examples of coverage kinds supported by Emma.
- PHPUnit: PHPUnit is a PHP programmers’ unit testing tool. PHP takes little chunks of code, known as units, and tests each individually. The tool also enables developers to assert that a system operates in a specific way using pre-defined assertion techniques.
These are just some of the unit testing tools accessible. There are more unit testing tools, notably for C and Java, but regardless of the language users utilize, users will find a unit testing tool to meet their needs.
Test-Driven Development (TDD) & Unit Testing
Test-driven development makes considerable use of testing frameworks when it comes to unit testing. To generate automated unit tests, a good unit test framework is used. Unit testing frameworks are not exclusive to TDD, although they are critical. We will go through a few of the benefits of TDD in the field of unit testing below:
- Prior to the code being written, tests are written.
- Make extensive use of testing frameworks.
- The applications’ classes are put to the test.
- It’s possible to integrate quickly and easily.
Why Unit Testing?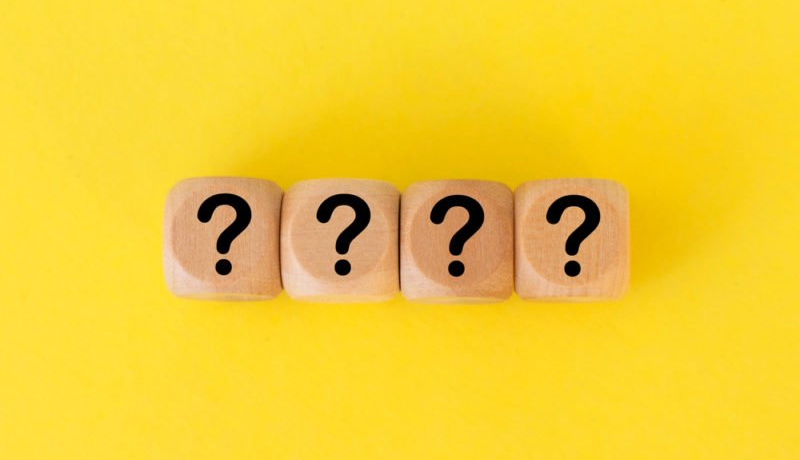
Since software developers want to save time by implementing limited unit testing, this is a misconception (testing myth) because insufficient unit testing leads to significant costs for defect correction during System Testing, Integration Testing, and even Beta Testing after the program has been built. Unit tests save time and money in the long run if proper unit testing is done early in the development process.
The following are the root causes for performing unit testing in software engineering:
- By discovering bugs early in the development cycle, unit tests save time and money (debugging).
- It helps developers understand the testing codebase and allows them to make quick changes.
- Well-written unit tests serve as project documentation.
- Unit tests make it easier to reuse code. Your tests and code should both be transferred to your new project. Adjust the code till the pass-code for the tests changes.
Unit Testing Myth
Myths are erroneous assumptions. Due to these assumptions, a vicious cycle emerges:
Unit testing does speed up development.
Integration Tests, programmers believe, will catch all mistakes and not run the unit test. Simple mistakes that could have been detected and repaired quickly in simple unit tests need a long period of time to trace and fix once the units have been combined.
Unit Testing Advantage And Disadvantages
Unit Testing Advantages
- The creation of unit tests provides a fundamental overview of the unit API test method for developers who want to discover what function a unit provides and how to use it.
- Unit testing enables the programmer to rewrite the unit of code later while ensuring that the module continues to function correctly (i.e., Regression testing). The unit testing tutorial is to develop test cases for all functions and methods so that any modifications that cause an issue can be smoothly discovered and rectified.
- We can test portions of the project without waiting for others to finish due to the modular structure of unit testing.
Unit Testing Disadvantages
- Expecting unit testing to catch every bug in a program is impractical. Also in the simplest programs, it is impossible to evaluate all possible execution routes.
- Unit testing, by definition, focuses on a single piece of code. Consequently, it is unable to detect system-wide or integration concerns.
- Unit testing should be performed alongside other sorts of tool testing.
Unit Testing Best Practices
Arrange, Act, Assert
Let us look at another type of unit test anatomy- this refers to the logical components of a decent unit test. Those components are “organize, act, affirm,” which is perhaps unsurprising considering the title of this section. The components are presented in their most basic form in the written test.
To grasp the true meaning of this, rely primarily on the scientific method. Consider your test as an experiment and your run as a hypothesis. To carry out this experiment, we must first gather all necessary materials. A calculator tester is instantiated. In other, more sophisticated instances, one may need to seed an object with variable values or invoke a specific constructor.
We take action now that the planning is complete. We use the added technique and capture the result in this example. The “act” reflects the unit testing show’s star. Everything that has gone before has led up to this point, and everything that follows amounts to retrospection. Finally, we assert. The assert idea in the unit test illustrates a broad category of behavior that you can’t leave out and still have a unit test. It is the hypothesis that is asserted. The essence of testing is asserting something.
One Assert Per Test Method
Contrary to popular belief, many assertions can reflect a single hypothesis. This means no test should ever include more than one assertion. However, your unit test suite should have a test to assert ratio of almost 1:1. Testing at the single-unit level, newbies frequently make the error of testing everything in one test method. After all, isn’t it true that more testing is better? This motivates them to obtain the most bang for their buck with each testable code by asserting a large number of points. However, keep in mind that hypothesis and experiment are two different things. Consider reading the test’s output in the test run. Even if you assert 20 things, there will only be one failure. How will you know what went wrong — which of your 20 claims failed?
Avoid Test Interdependence
Each test should be responsible for its setup and dismantling. The test runner will run your legacy code in any sequence it sees fit, and depending on the runner you’re using (advanced subject), it may even run them in parallel. As a result, you can’t rely on the test suite or the class you’re testing to keep state between tests. However, this will not always be evident to you. For example, if you have two tests, the test runner may run them in the same order each time. You may start to rely on this after being lulled into a false feeling of security. When you add a third test a few weeks later, it throws off balance, and one of your end tests begins to fail intermittently due to the ordering. This will perplex and enrage you. At all costs, avoid interdependence.
Keep It Short, Sweet, and Visible
Refrain from abstracting test setup (the “arrange”) to other classes, especially from abstracting it into a base class. The logic is straightforward. When a test fails, users want to know why it failed. As a result, you want a test where all of the setup logic is visible at a look. You’ll have a faulty treasure hunt on your hands if you have logic spread over the class or in multiple classrooms. That’s terrible enough in production code, but tests are intended to help. Make it as straightforward as possible for yourself.
Recognize Test Setup Pain as a Smell
You’ll learn to write unit tests on toy codebases when you start. Before you hit this, you’ll need some real-world experience and a few notches on your belt, but you’ll get there. Stop what you’re doing if your unit test’s “setup” phase becomes too difficult. One of the most underappreciated benefits of unit tests is that they provide good feedback on your code’s design, particularly its modularity. You have a design problem if you have to work hard to put up a class and method to test it. Brittle tests are created when hefty tests are set up. Tests, like production code, have a maintenance weight. As a result, you should avoid clumsy tests like the plague – they’ll break and make you and everyone else despise them. Instead, users should take a close look at their design.
Add Them to the Build
This is perhaps the most significant best practice. Since you’re new to unit testing, get started on this one right away, even if you only have one test in your codebase. If your team uses a continuous integration test build, include the execution of your new unit test suite in the build. In case any of the tests fail, the build will fail. If unit test failures do not halt your team’s development, when the pressure to deliver mounts, your team will soon ignore the failures. It’s a question of when not if. It takes time to learn the unit tested and much longer to master it. At first, achieving mastery will seem impossible, but if you don’t go all in, you’ll never get there.
Relevant Information On Unit Testing
The unit-testing process is built into an IDE (or via extensions) so that it runs the tests with each compile. There are multiple frameworks available to aid in the production of unit tests (and indeed mock/ stub objects)
Quality assurance in unit testing
Software quality assurance is described as a technique for ensuring the quality of software goods or services given to clients by a company. Quality assurance deals with making the software development process more effective and efficient in accordance with the quality control standards for software products. Quality Assurance Testing is a common term for Quality Assurance.
Summary
- Unit testing is a software testing method in which individual program units or components are tested.
- Unit testing may be somewhat time-consuming. It might be complex or simple based on the application being tested and the testing methodologies, tools, and philosophies employed. On some level, unit testing is always needed. That is undeniable.