What Are Unit Tests In Programming?
Unit testing is a kind of Testing in which individual units or functions of software testing. The primary purpose is to test each unit or operation. A team is the often smallest testable part of an application. It is mainly one or a few inputs and produces a single output. In procedural programming, an individual unit is referred to as an individual program or individual function; on the other hand, object-oriented programming languages have Base/Superclass, abstract class, Derived/Child class. Unit test frameworks, stubs, drivers, and mocks /fake objects are used in Unit Testing. It works based on a White box technique.
In V Model SDLC (Software development life cycle), STLC, this testing is the first level of Testing done before integration testing. Unit testing is a WhiteBox testing technique usually performed by the developer. Due to other reasons such as time crunch or reluctance of developers to test, in a practical world, QA engineers also do unit testing.
With Unit Testing, Enterprises can:
- Improve Quality assurance of Code
- Build Reusable and Reliable Code
- Simplify Documentation
- Enable Seamless Integration
Table of Content:
- How does unit testing work?
- How to do unit testing?
- Why unit testing?
- What are the benefits of unit testing?
- What Do Unit Tests Look Like?
- The best unit testing tools
- Test-Driven Development (TDD) & Unit Testing
- Unit testing myths
- What can I do with them?
- Conclusion
How Does Unit Testing Work?
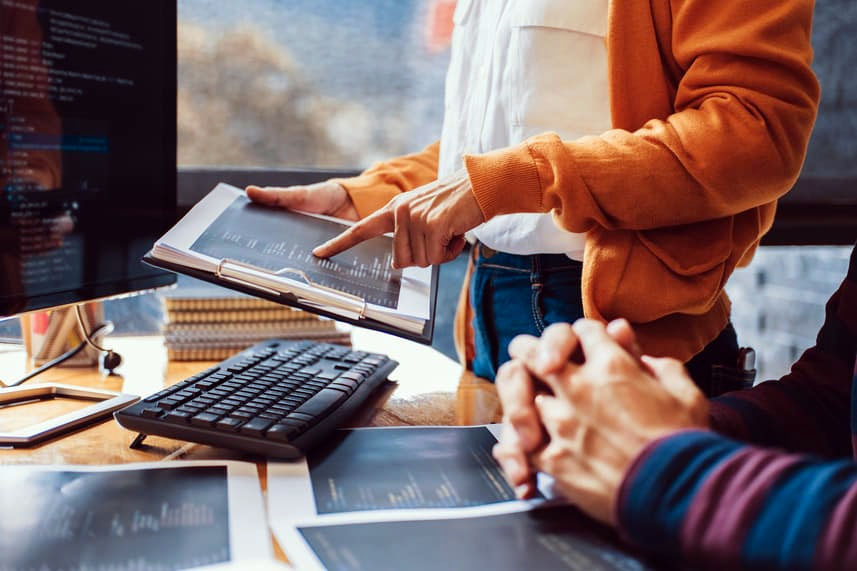
To do this, mocks are required. Is there a need for mocks to do Testing on functions? You should; without creating mocks, functions cannot be unit tested. Testing works based on mock objects. Generally, these mock objects work to fill in for missing parts in your program. Such as; there might be a function that needs variables or objects that have not been created yet. To test the function, mock objects were created. In such scenarios, mock objects fill missing parts.
White-Box testing
It’s referred to as glass box testing or transparent Testing or Integrity testing. In this kind of testing, the tester is usually conscious of internal functionality. It is the internal structure of an item or function to be tested is that is justify unknown to them.
Black-Box testing
It is a type of Testing; the tester is usually not made aware of the internal functionality of a system. It is the internal structure of the function to be tested. It is unknown.
Gray-Box Testing
It’s referred to as semi-transparent Testing. It is a combination of White Box and Balck Box testing. This combination is the type of testing in which the tester is aware of the internal functionality of a method or unit, however, not at a more profound level like white box testing. In this, the user is partially aware of the internal functionality of a system. Various types of Testing covered under a Gray box testing is mentioned as follows:
- Matrix testing.
- Pattern Testing.
- Orthogonal Pattern testing.
- Regression Testing.
How To Do Unit Testing?
To successfully do testing, developers write a section of code so that they can test a specific function in the software application. Then the developers can also isolate this function to test it more rigorously, revealing unnecessary dependencies that occur between the function being tested and other units, so the dependencies are eliminated where necessary. Developers generally utilize the UnitTest framework to develop automated test cases for unit testing.
Type of Unit Testing
- Manual
- Automated
This testing is commonly automated; however, it may still be performed manually. Software Engineering does not usually favor one over the other; however, automation is preferred. A manual testing approach to unit testing is likely to employ a step-by-step instructional document.
Under the automated approach
A developer writes a code section in the application to test the function. They would later comment and remove the test codes when deployed.
A developer could also isolate the function and test it more rigorously. This is likely a more thorough unit testing practice involving copying and pasting code to its testing environment than its natural environment. Isolating the code helps reveal unnecessary dependencies that are present between the code being tested and any other units or data spaces present in the product. These dependencies can then be eliminated.
A coder generally utilizes a UnitTest Framework to develop automated test cases. Utilizing an automation framework, the developer can code criteria into the test to verify the correctness of the code. During the execution of these cases, the framework can log failing cases. Many frameworks are designed to automatically flag and report these failed test cases in summary. This depends on the severity of a failure; the framework may halt subsequent testing.
The workflow of Testing is as listed below:
- Create Test Cases
- Review/Rework
- Baseline
- Execute Test Cases.
Why Unit Testing?
Unit Testing is essential because software developers sometimes try saving time doing minimal testing. This is clearly a myth because inappropriate testing leads to high-cost defect fixing on your part during System Testing, Integration Testing, and even Beta Testing after the application is built. Proper unit testing should be done in early development to save time and money.
What are the benefits of Unit Testing?
With Unit Tests, you can reveal a basic understanding of API units or functions to understand the code’s functionality. It is a means for you to write cases for all the functions and methods so that whenever there is a change that causes a fault, the bug is quickly identified and then fixed. A modular approach is usually followed in functional Testing, single-single functionality, or part of the source code tested without waiting for the other code base to be completed.
Why Unit Testing Matters?
Unit testing helps you to maintain and change the code. It is a type of Testing that helps you by increasing the speed of Testing. If you have good unit test cases written for code and run every time code changes, any defect is revealed after code changes. Codes should be more reusable; in a modular way, more testing will be possible. Writing test cases takes time, but this can be compensated by less time to run the tests.
Having unit tests is more reliable, and the development cycle will be faster in the long run too. It makes debugging easy for you. When a test fails, only the latest changes need to be debugged. When you are Testing at a higher level or higher phases, changes made over days/ weeks/months will need to be scanned or detected. However, the cost to fix detected bugs during Testing is lesser than the defects at higher levels. Compare the cost of repairing a defect when a bug is detected in a unit testing phase with the same bug detected in User Acceptance Testing. This is not something to look forward to; the effort required to find and fix defects found during Testing is less than the effort needed to correct defects found during System Testing or Acceptance Testing.
How to adopt Unit Testing?
Unit test cases are automated but still created manually; for you to do Testing with an automated approach, the following steps will need to be considered – You can write another section of code in your application to test a function. To do this, unit test cases were created. Later on, this section of code was finally removed to test code when an application is complete or when an application is working.
Isolation of a code – This Isolate function is put to the test more rigorously. The code can do Automated Unit Testing in a better way. Isolating functions/code aids in Testing in a good way. Because it helps to reveal dependencies between functions of code, you can use the Test framework for developing automated test cases. During cases, the framework display logs for failed cases. Nowadays, many frameworks automatically flag and report failed cases in summary. Depending upon failure, failure may halt subsequent testing.
What Do Unit Tests Look Like?
Unit Tests can be almost anything you want them to be – a line of code, a method, or a class. Generally, though, more minor is better. Smaller tests give you a more granular view of your code’s performance. The practical aspect is that when you test minimal units, your tests can be run fast, for instance, a thousand tests in a second fast.
Consider the sample code below:
def divider (a, b)
return a/b
end
Using Ruby, those small tests might look something like this:
class smallTest < MiniTest::Unit::testCase
def tiny_test
@a=9
@b=3
assert_equal(3, divider(a, b))
end
end
The example provided is overly simple, but it gives you an idea of what I mean by small. Small tests also benefit from making it harder to cross-systems — from code into a database or 3rd party system. Strictly speaking, there isn’t anything incorrect with crossing systems, but there are consequences like gradually slowing your tests.
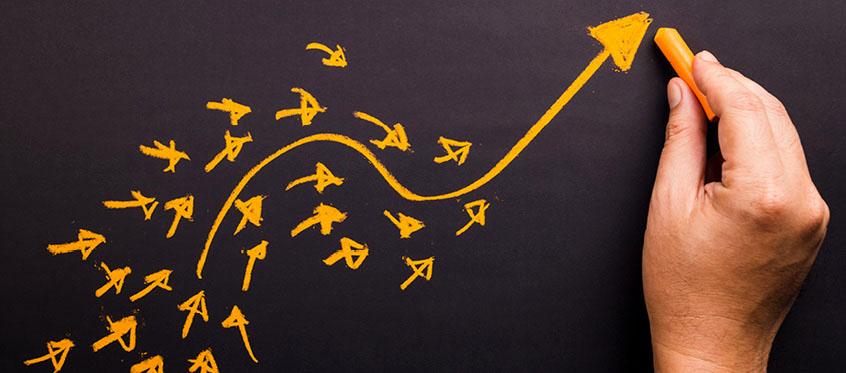
The Best Practices of Unit Testing
- You do not need to create test cases for every condition possible. Instead of that, you should focus on tests that impact the entire system’s behavior. Before fixing a bug, you should write a test to expose the defects.
- If the bug will re-occur if not appropriately cached.
- Test suites should be more comprehensive.
- Write cases before fixing the defect.
- Write cases independent of each other. For example, if a coding class depends upon a database, you should not write a case that is meant to interact with a database to test a class. Instead, create an abstract interface around that database connection and implement an interface using a mock object.
- The crucial thing for Unit Testing is to cover all the paths; it is also required to pay attention to loop conditions.
- You can write cases to verify behavior, additionally, write cases to ensure the performance of the code.
- You can also execute cases continuously and frequently.
The Best Unit Testing Tools
Tool | Characteristic |
Jtest | Parasoft Jtest is an IDE plugin that generally leverages an open-source framework (JUnit,mockito, PowerMock, and Spring) with guided and provides one-click actions to create, scale, and maintain unit tests. Automating the time-consuming aspects of Testing focuses on business logic and creates more meaningful test suits. |
JUnit | JUnit is a tool that tests data first then inserts it into a piece of code. It is free to use for the Java programming language. It also provides you with support for assertions to identify test methods. |
NUnit | It is used as a Testing framework, and this tool is used for all Net languages, for example python. It is also an open-source tool to write scripts manually. NUnit supports data-driven tests run in parallel. |
PHPUnit | PHP Unit is a Testing tool for PHP language. It takes small portions of code called units and tests each unit separately. This testing tool uses pre-defined assertion methods to assert that the system should behave specifically. |
EMMA | It is an open-source toolkit used for analyzing and reporting code written in Java. EMMA supports coverage types like method, basic block, and line. It is also a Java-based tool. |
JMockit | JMockit is a code coverage tool. It is an open-source testing tool. It helps in mocking API test with recording and verification of syntax. It is a tool that offers you line coverage, test data coverage, and path coverage. |
Test-Driven Development (TDD) & Unit Testing
Unit testing in TDD will involve extensive use of testing frameworks. A unit test framework is used to create automated unit tests. While unit testing frameworks are not unique to TDD, they are essential. Below we have highlighted some of what TDD brings to the world of unit testing:
- Rely heavily on testing frameworks
- All classes in the applications are tested
- Tests are written before the code
- Quick and easy integration is made possible
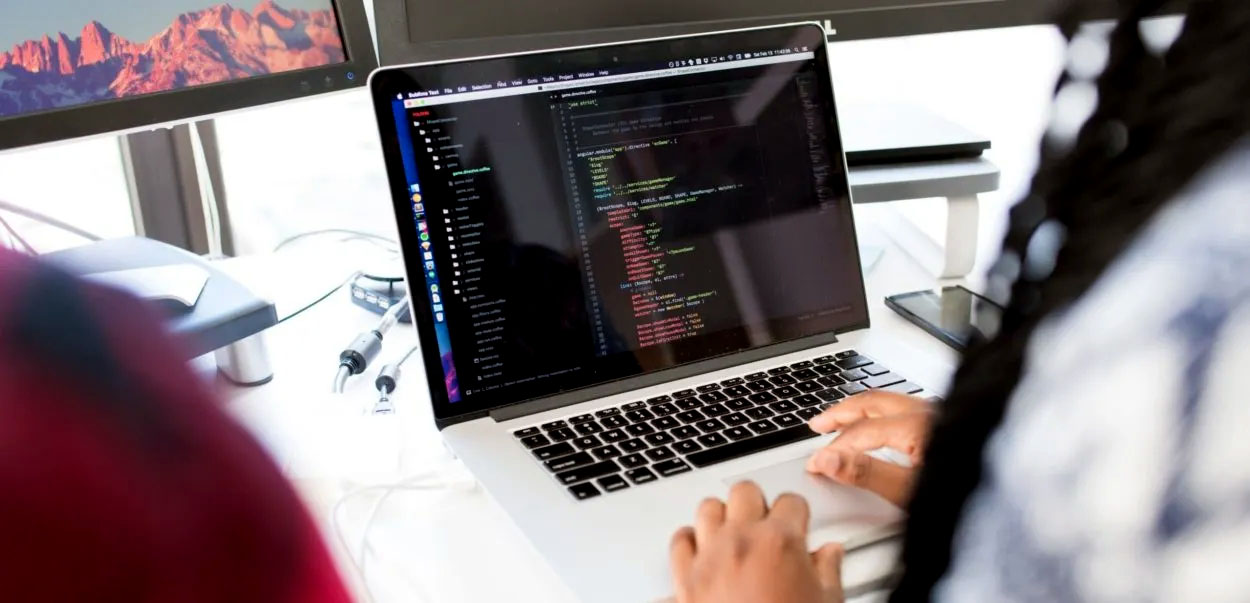
What Can I Do With Them?
Hammers are great tools to help you with various jobs — opening car windows or turning off alarm clocks. However, there are especially well suited to putting nails through hard surfaces. Unit tests are similar. They can do many different things; they should probably only do a few.
Test-Driven Development
Test-Driven Development, also called TDD, is a code design technique where the programmer writes a test before any production code is done and then writes the code that will make that test pass. The idea is that you gain a tiny bit of assurance that you have from that initial test you do; the programmer can feel free to refactor and refactor some more so as to get the cleanest code that they know how to write. The idea is rather simple, but like most simple things, the execution is complex. TDD requires an entirely different mindset from what most developers are used to, as well as the tenacity required to deal with a learning curve that may actually slow you down at first.
Helps you in Checking Your Work
TDD is not new, but at this point, it is still mainly for the go-getters. Most of us are checking our work. After you have written the production code, writing unit tests is the more traditional way of doing things, but it is no less valuable. It may also be something you are more familiar with if you have been in a math class any time in the past decade.
After your work has been checked and it is clear that the code is doing what you think it is doing, it is normal that the value of the unit tests changes a little bit. Tests that can be efficiently run with every build of your product act as change detection, notifying you or your team when code changes in unexpected ways.
Helps with the Code Documentation
Code documentation is often a drag, and it shows mostly how little code documentation gets written. Unit testing can help make the documentation burden a little easier by encouraging better coding practices and leaving behind code pieces that describe what your product is doing. Rather than constantly feeding the documentation beast with a code change, you will be updating a system of checks that is working for you or your team instead.
Avoid the danger zone.
There are minimal uses of unit testing techniques that you will want to avoid when possible—creating integration tests that You can do cross-system borders and touch databases or 3rd party systems quickly results in a test suite that takes a long time to run with each added test. There are several test frameworks out there that specialize in higher-level Testing. When you want to test larger pieces of your product at a time, you might want to investigate those other frameworks. One other risky area is an end to end tests. These usually require you to order carefully, dependencies on other tests, and a detailed setup to get your system in a particular ‘test ready’ state. Much like integration testing, there are several automation tools to choose from made just for this purpose.
You are definitely capable of doing these things with unit frameworks, but it might quickly become more work than it is worth.
Conclusion
This Testing is only required when testing each function independently. This type of Testing helps you save time and cost. If the same issue is faced at Acceptance Testing, then, in that case, the price will be more than the cost of Unit Testing. At some points to do testing, there is a requirement that you must create mock objects that can satisfy or fulfill the dependency of a method or a function in the system. If a function is dependent on another function and another function is still not created, then the mock function object is used.